Math 152: Intro to Mathematical Software
2017-02-27
Kiran Kedlaya; University of California, San Diego
adapted from lectures by William Stein, University of Washington
Lecture 20: Numpy, Matplotlib, and Scipy (part 1): Overviews
Announcements:
Modified office hours this week: mine are today 3-4; Zonglin's are Tuesday 10-12.
As usual, homework due Tuesday 8pm. Peer grading due Thursday 8pm.
Advance warning: Peter and Zonglin and I will simultaneously be away at a conference during the first half of week 10 (the week starting March 13). We will thus have a modified schedule for that week, with the problem set and peer grading due later than usual. Details to follow. (Remember, there is no final exam for this course.)
10 minute intros to numpy, matplotlib and scipy.
Numpy, Matplotlib, and Scipy are (along with Cython) the foundation of the Scientific Python Stack.
Pretty much everything in the world of Python "data science" depends on them.
The community is unified around them as the foundation on which to build.
All are very polished and heavily used at this point.
Written in mostly C, C++ and some Fortran (scipy). Very highly optimized for speed. Lots of "vectorized operations".
We'll take a quick look at some tutorials for each of these packages. Later in the week, we'll demonstrate these in more details within SMC.
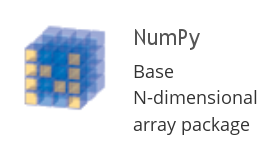
You should spend 2 hours and go through the Numpy Tutorial.

You should spend an hour and go through this Matplotlib tutorial.
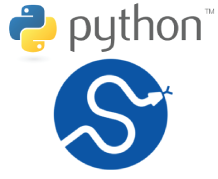
You should spend several hours on the Scipy tutorial.