Real-time collaboration for Jupyter Notebooks, Linux Terminals, LaTeX, VS Code, R IDE, and more,
all in one place. Commercial Alternative to JupyterHub.
Real-time collaboration for Jupyter Notebooks, Linux Terminals, LaTeX, VS Code, R IDE, and more,
all in one place. Commercial Alternative to JupyterHub.
Path: blob/main/06. Databases and SQL for Data Science with Python/04. Accessing Databases using Python/02. Query a DB2 Database using Python.ipynb
Views: 5156
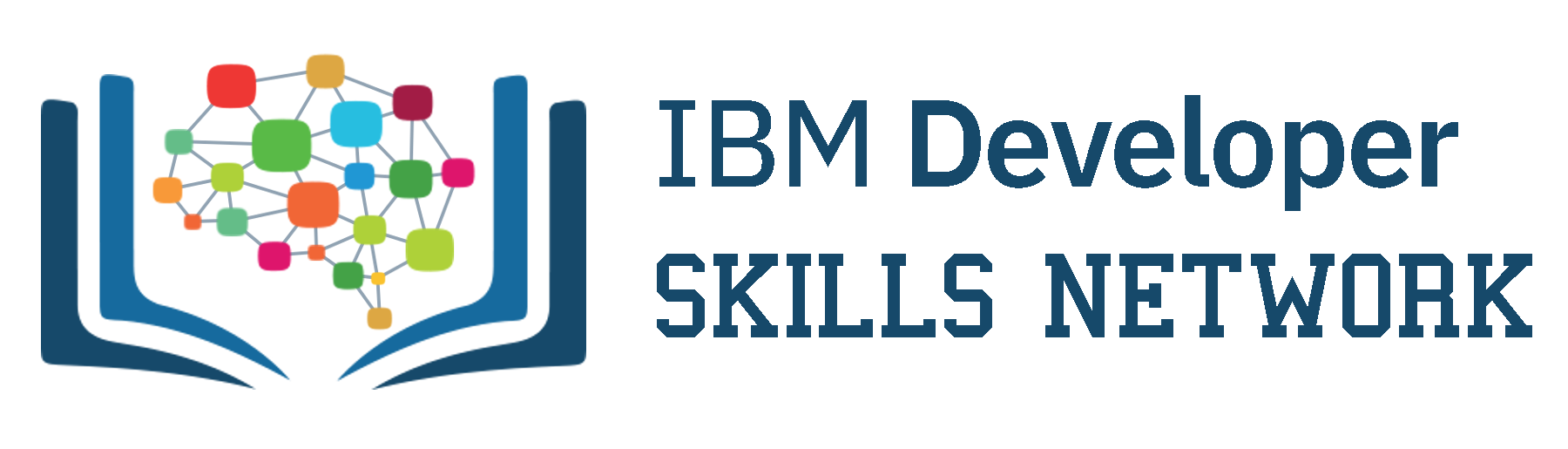
Query a DB2 Database using Python
Objectives
After completing this lab you will be able to:
Create a table
Insert data into the table
Query data from the table
Retrieve the result set into a pandas dataframe
Close the database connection
Notice: Please follow the instructions given in the first Lab of this course to Create a database service instance of Db2 on Cloud.
Task 1: Import the ibm_db
Python library
The ibm_db
API provides a variety of useful Python functions for accessing and manipulating data in an IBM® data server database, including functions for connecting to a database, preparing and issuing SQL statements, fetching rows from result sets, calling stored procedures, committing and rolling back transactions, handling errors, and retrieving metadata.
We import the ibm_db library into our Python Application
When the command above completes, the ibm_db
library is loaded in your notebook.
Task 2: Identify the database connection credentials
Connecting to dashDB or DB2 database requires the following information:
Driver Name
Database name
Host DNS name or IP address
Host port
Connection protocol
User ID
User Password
Notice: To obtain credentials please refer to the instructions given in the first Lab of this course
Now enter your database credentials below
Replace the placeholder values in angular brackets <> below with your actual database credentials
e.g. replace "database" with "BLUDB"
Task 3: Create the database connection
Ibm_db API uses the IBM Data Server Driver for ODBC and CLI APIs to connect to IBM DB2 and Informix.
Create the database connection
Task 4: Create a table in the database
In this step we will create a table in the database with following details:
---------------------------------------------------------------------------
Exception Traceback (most recent call last)
<ipython-input-4-83413676a2ca> in <module>
3
4 #Now execute the drop statment
----> 5 dropStmt = ibm_db.exec_immediate(conn, dropQuery)
SQLCODE=-204ion: [IBM][CLI Driver][DB2/LINUXX8664] SQL0204N "KFM42587.INSTRUCTOR" is an undefined name. SQLSTATE=42704
Dont worry if you get this error:
If you see an exception/error similar to the following, indicating that INSTRUCTOR is an undefined name, that's okay. It just implies that the INSTRUCTOR table does not exist in the table - which would be the case if you had not created it previously.
Exception: [IBM][CLI Driver][DB2/LINUXX8664] SQL0204N "ABC12345.INSTRUCTOR" is an undefined name. SQLSTATE=42704 SQLCODE=-204
Task 5: Insert data into the table
In this step we will insert some rows of data into the table.
The INSTRUCTOR table we created in the previous step contains 3 rows of data:
We will start by inserting just the first row of data, i.e. for instructor Rav Ahuja
Now use a single query to insert the remaining two rows of data
Task 6: Query data in the table
In this step we will retrieve data we inserted into the INSTRUCTOR table.
Bonus: now write and execute an update statement that changes the Rav's CITY to MOOSETOWN
Task 7: Retrieve data into Pandas
In this step we will retrieve the contents of the INSTRUCTOR table into a Pandas dataframe
Once the data is in a Pandas dataframe, you can do the typical pandas operations on it.
For example you can use the shape method to see how many rows and columns are in the dataframe
Task 8: Close the Connection
Free all resources by closing the connection. Remember that it is always important to close connections so that we can avoid unused connections taking up resources.