Real-time collaboration for Jupyter Notebooks, Linux Terminals, LaTeX, VS Code, R IDE, and more,
all in one place. Commercial Alternative to JupyterHub.
Real-time collaboration for Jupyter Notebooks, Linux Terminals, LaTeX, VS Code, R IDE, and more,
all in one place. Commercial Alternative to JupyterHub.
Path: blob/main/07. Data Analysis with Python/01. Importing Datasets/01. Importing Datasets.ipynb
Views: 5156
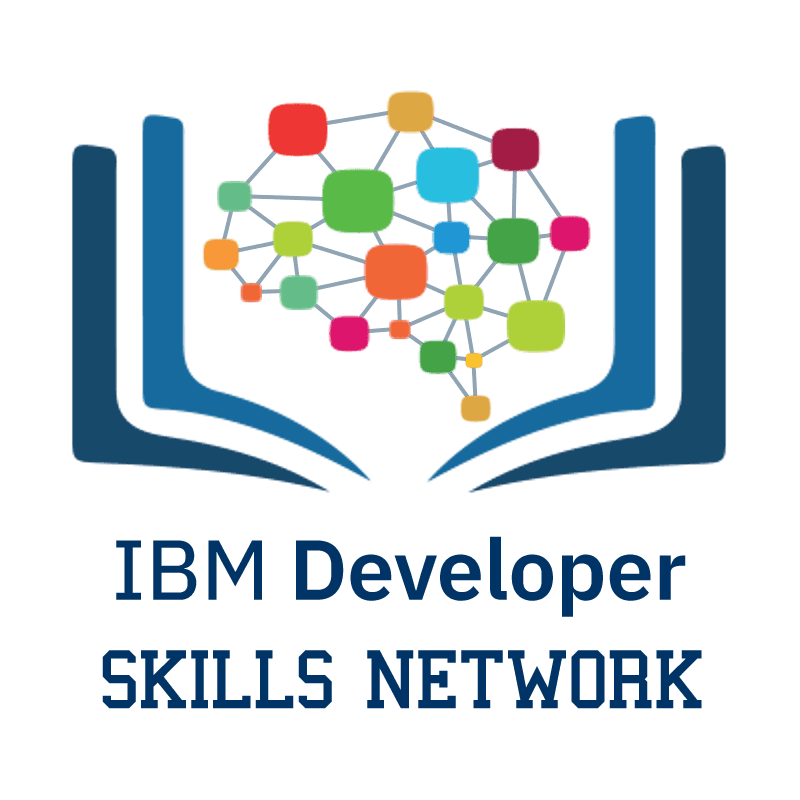
Introduction Notebook
Objectives
After completing this lab you will be able to:
Acquire data in various ways
Obtain insights from data with Pandas library
Data Acquisition
There are various formats for a dataset: .csv, .json, .xlsx etc. The dataset can be stored in different places, on your local machine or sometimes online.
In this section, you will learn how to load a dataset into our Jupyter Notebook.
In our case, the Automobile Dataset is an online source, and it is in a CSV (comma separated value) format. Let's use this dataset as an example to practice data reading.
- Data source: https://archive.ics.uci.edu/ml/machine-learning-databases/autos/imports-85.data
- Data type: csv
Read Data
We use pandas.read_csv()
function to read the csv file. In the brackets, we put the file path along with a quotation mark so that pandas will read the file into a dataframe from that address. The file path can be either an URL or your local file address.
Because the data does not include headers, we can add an argument headers = None
inside the read_csv()
method so that pandas will not automatically set the first row as a header.
You can also assign the dataset to any variable you create.
After reading the dataset, we can use the dataframe.head(n)
method to check the top n rows of the dataframe, where n is an integer. Contrary to dataframe.head(n)
, dataframe.tail(n)
will show you the bottom n rows of the dataframe.
Question #1:
Check the bottom 10 rows of data frame "df".Add Headers
Take a look at our dataset. Pandas automatically set the header with an integer starting from 0.
To better describe our data, we can introduce a header. This information is available at: https://archive.ics.uci.edu/ml/datasets/Automobile.
Thus, we have to add headers manually.
First, we create a list "headers" that include all column names in order.
Then, we use dataframe.columns = headers
to replace the headers with the list we created.
We replace headers and recheck our dataframe:
We need to replace the "?" symbol with NaN so the dropna() can remove the missing values:
We can drop missing values along the column "price" as follows:
Now, we have successfully read the raw dataset and added the correct headers into the dataframe.
Question #2:
Find the name of the columns of the dataframe.Save Dataset
Correspondingly, Pandas enables us to save the dataset to csv. By using the dataframe.to_csv()
method, you can add the file path and name along with quotation marks in the brackets.
For example, if you would save the dataframe df as automobile.csv to your local machine, you may use the syntax below, where index = False
means the row names will not be written.
We can also read and save other file formats. We can use similar functions like pd.read_csv()
and df.to_csv()
for other data formats. The functions are listed in the following table:
Read/Save Other Data Formats
Data Formate | Read | Save |
---|---|---|
csv | pd.read_csv() | df.to_csv() |
json | pd.read_json() | df.to_json() |
excel | pd.read_excel() | df.to_excel() |
hdf | pd.read_hdf() | df.to_hdf() |
sql | pd.read_sql() | df.to_sql() |
... | ... | ... |
Basic Insight of Dataset
After reading data into Pandas dataframe, it is time for us to explore the dataset.
There are several ways to obtain essential insights of the data to help us better understand our dataset.
Data Types
Data has a variety of types.
The main types stored in Pandas dataframes are object, float, int, bool and datetime64. In order to better learn about each attribute, it is always good for us to know the data type of each column. In Pandas:
A series with the data type of each column is returned.
As shown above, it is clear to see that the data type of "symboling" and "curb-weight" are int64
, "normalized-losses" is object
, and "wheel-base" is float64
, etc.
These data types can be changed; we will learn how to accomplish this in a later module.
Describe
If we would like to get a statistical summary of each column e.g. count, column mean value, column standard deviation, etc., we use the describe method:This method will provide various summary statistics, excluding NaN
(Not a Number) values.
Now it provides the statistical summary of all the columns, including object-typed attributes.
We can now see how many unique values there, which one is the top value and the frequency of top value in the object-typed columns.
Some values in the table above show as "NaN". This is because those numbers are not available regarding a particular column type.
Question #3:
You can select the columns of a dataframe by indicating the name of each column. For example, you can select the three columns as follows:
dataframe[[' column 1 ',column 2', 'column 3']]
Where "column" is the name of the column, you can apply the method ".describe()" to get the statistics of those columns as follows:
dataframe[[' column 1 ',column 2', 'column 3'] ].describe()
Apply the method to ".describe()" to the columns 'length' and 'compression-ratio'.
Info
Another method you can use to check your dataset is dataframe.info():It provides a concise summary of your DataFrame.
This method prints information about a DataFrame including the index dtype and columns, non-null values and memory usage.