Real-time collaboration for Jupyter Notebooks, Linux Terminals, LaTeX, VS Code, R IDE, and more,
all in one place. Commercial Alternative to JupyterHub.
Real-time collaboration for Jupyter Notebooks, Linux Terminals, LaTeX, VS Code, R IDE, and more,
all in one place. Commercial Alternative to JupyterHub.
Path: blob/main/08. Data Visualization with Python/10. Dash Interactivity.ipynb
Views: 5156
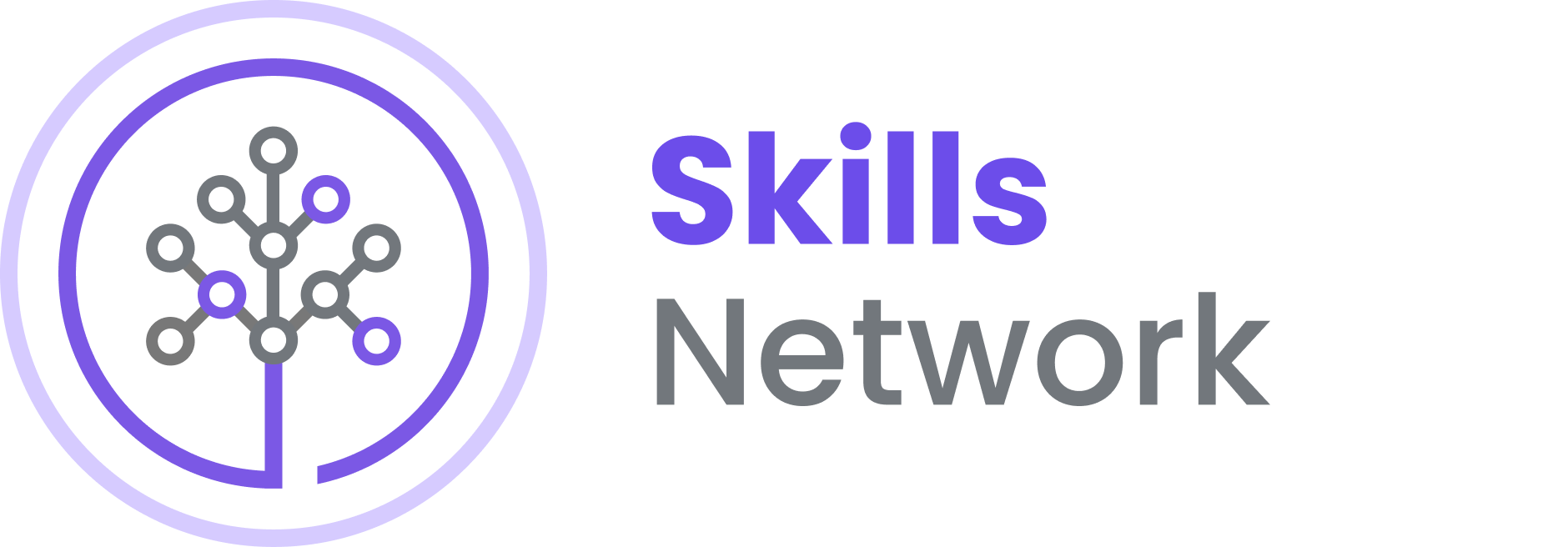
Objectives
In this lab, you will work on Dash Callbacks. When this notebook is run successfully, the app will be run on localhost.
Dataset Used
Airline Reporting Carrier On-Time Performance dataset from Data Asset eXchange
Lab Question
Extract average monthly arrival delay time and see how it changes over the year.
Dash Application Creation
Todo for the lab question
Import required libraries, read the airline data, and create an application layout
Add title to the dashboard using HTML H1 component
Add a HTML division and core text input component inside the division. Provide an input component id and a default value to the component.
Add a HTML dividion and core graph component. Provide a graph component id.
Add callback decorator and provide input and output parameters.
Define callback function, perform computation to extract required information, create the graph, and update the layout.
Run the app
Hints
General examples can be found here.
For step 1 (only review), this is very specific to running app from Jupyerlab.
For Jupyterlab,we will be using
jupyter-dash
library. Addingfrom jupyter_dash import JupyterDash
import statement.Instead of creating dash application using
app = dash.Dash()
, we will be usingapp = JupyterDash(__name__)
.Use pandas to read the airline data.
For step 2,
Title as
Airline Performance Dashboard
Use
style
parameter and make the title center aligned, with color code#503D36
, and font-size as 40. CheckMore about HTML
section here.
For step 3,
For step 4,
Core graph component and assign id as
line-plot
.
For 5 and 6,
Basic callback
App Skeleton
* Running on http://127.0.0.1:8050/ (Press CTRL+C to quit)
127.0.0.1 - - [13/Feb/2022 15:27:25] "GET / HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:25] "GET /_dash-component-suites/dash/deps/[email protected]_1_0m1644765086.12.1.min.js HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:25] "GET /_dash-component-suites/dash/deps/[email protected]_1_0m1644765086.14.0.min.js HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:25] "GET /_dash-component-suites/dash/deps/[email protected]_1_0m1644765086.14.0.min.js HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:25] "GET /_dash-component-suites/dash/deps/[email protected]_1_0m1644765086.7.2.min.js HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:25] "GET /_dash-component-suites/dash/dash-renderer/build/dash_renderer.v2_1_0m1644765085.min.js HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:25] "GET /_dash-component-suites/dash/dcc/dash_core_components.v2_1_0m1644765086.js HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:25] "GET /_dash-component-suites/dash/dcc/dash_core_components-shared.v2_1_0m1644765086.js HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:25] "GET /_dash-component-suites/dash/html/dash_html_components.v2_0_1m1644765086.min.js HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:25] "GET /_dash-component-suites/dash/dash_table/bundle.v5_1_0m1644765085.js HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:26] "GET /_dash-layout HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:26] "GET /_dash-dependencies HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:26] "GET /_favicon.ico?v=2.1.0 HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:26] "GET /_dash-component-suites/dash/dcc/async-graph.js HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:26] "GET /_dash-component-suites/dash/dcc/async-plotlyjs.js HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:27] "POST /_dash-update-component HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:28] "GET /_favicon.ico?v=2.1.0 HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:31] "POST /_dash-update-component HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:31] "POST /_dash-update-component HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:32] "POST /_dash-update-component HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:32] "POST /_dash-update-component HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:35] "POST /_dash-update-component HTTP/1.1" 200 -
127.0.0.1 - - [13/Feb/2022 15:27:35] "POST /_dash-update-component HTTP/1.1" 200 -