Real-time collaboration for Jupyter Notebooks, Linux Terminals, LaTeX, VS Code, R IDE, and more,
all in one place. Commercial Alternative to JupyterHub.
Real-time collaboration for Jupyter Notebooks, Linux Terminals, LaTeX, VS Code, R IDE, and more,
all in one place. Commercial Alternative to JupyterHub.
Path: blob/main/09. Machine Learning with Python/02. Regression/04. Non-Linear Regression.ipynb
Views: 4598
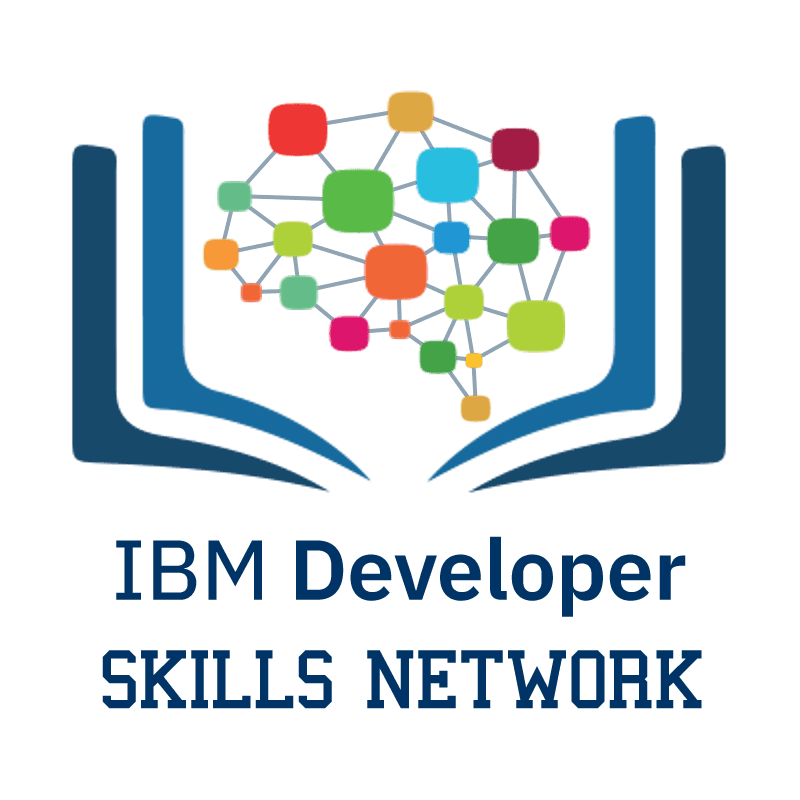
Non Linear Regression Analysis
Objectives
After completing this lab you will be able to:
Differentiate between linear and non-linear regression
Use non-linear regression model in Python
If the data shows a curvy trend, then linear regression will not produce very accurate results when compared to a non-linear regression since linear regression presumes that the data is linear. Let's learn about non linear regressions and apply an example in python. In this notebook, we fit a non-linear model to the datapoints corrensponding to China's GDP from 1960 to 2014.
Importing required libraries
Although linear regression can do a great job at modeling some datasets, it cannot be used for all datasets. First recall how linear regression, models a dataset. It models the linear relationship between a dependent variable y and the independent variables x. It has a simple equation, of degree 1, for example y = + 3.
Non-linear regression is a method to model the non-linear relationship between the independent variables and the dependent variable . Essentially any relationship that is not linear can be termed as non-linear, and is usually represented by the polynomial of degrees (maximum power of ). For example:
$$\ y = a x^3 + b x^2 + c x + d \$$Non-linear functions can have elements like exponentials, logarithms, fractions, and so on. For example:
We can have a function that's even more complicated such as :
Let's take a look at a cubic function's graph.
As you can see, this function has and as independent variables. Also, the graphic of this function is not a straight line over the 2D plane. So this is a non-linear function.
Some other types of non-linear functions are:
Quadratic
Exponential
An exponential function with base c is defined by where b ≠0, c > 0 , c ≠1, and x is any real number. The base, c, is constant and the exponent, x, is a variable.
Logarithmic
The response is a results of applying the logarithmic map from the input to the output . It is one of the simplest form of log(): i.e.
Please consider that instead of , we can use , which can be a polynomial representation of the values. In general form it would be written as
Sigmoidal/Logistic
For an example, we're going to try and fit a non-linear model to the datapoints corresponding to China's GDP from 1960 to 2014. We download a dataset with two columns, the first, a year between 1960 and 2014, the second, China's corresponding annual gross domestic income in US dollars for that year.
Plotting the Dataset
This is what the datapoints look like. It kind of looks like an either logistic or exponential function. The growth starts off slow, then from 2005 on forward, the growth is very significant. And finally, it decelerates slightly in the 2010s.
Choosing a model
From an initial look at the plot, we determine that the logistic function could be a good approximation, since it has the property of starting with a slow growth, increasing growth in the middle, and then decreasing again at the end; as illustrated below:
The formula for the logistic function is the following:
: Controls the curve's steepness,
: Slides the curve on the x-axis.
Building The Model
Now, let's build our regression model and initialize its parameters.
Lets look at a sample sigmoid line that might fit with the data:
Our task here is to find the best parameters for our model. Lets first normalize our x and y:
How we find the best parameters for our fit line?
we can use curve_fit which uses non-linear least squares to fit our sigmoid function, to data. Optimize values for the parameters so that the sum of the squared residuals of sigmoid(xdata, *popt) - ydata is minimized.
popt are our optimized parameters.
Now we plot our resulting regression model.
Practice
Can you calculate what is the accuracy of our model?