Real-time collaboration for Jupyter Notebooks, Linux Terminals, LaTeX, VS Code, R IDE, and more,
all in one place. Commercial Alternative to JupyterHub.
Real-time collaboration for Jupyter Notebooks, Linux Terminals, LaTeX, VS Code, R IDE, and more,
all in one place. Commercial Alternative to JupyterHub.
Path: blob/main/10. Applied Data Science Capstone/04. Interactive Visual Analytics/04. Interactive Visual Analytics - Folium.ipynb
Views: 4598

Launch Sites Locations Analysis with Folium
Estimated time needed: 40 minutes
The launch success rate may depend on many factors such as payload mass, orbit type, and so on. It may also depend on the location and proximities of a launch site, i.e., the initial position of rocket trajectories. Finding an optimal location for building a launch site certainly involves many factors and hopefully we could discover some of the factors by analyzing the existing launch site locations.
In the previous exploratory data analysis labs, you have visualized the SpaceX launch dataset using matplotlib
and seaborn
and discovered some preliminary correlations between the launch site and success rates. In this lab, you will be performing more interactive visual analytics using Folium
.
Objectives
This lab contains the following tasks:
TASK 1: Mark all launch sites on a map
TASK 2: Mark the success/failed launches for each site on the map
TASK 3: Calculate the distances between a launch site to its proximities
After completed the above tasks, you should be able to find some geographical patterns about launch sites.
Let's first import required Python packages for this lab:
If you need to refresh your memory about folium, you may download and refer to this previous folium lab:
Task 1: Mark all launch sites on a map
First, let's try to add each site's location on a map using site's latitude and longitude coordinates
The following dataset with the name spacex_launch_geo.csv
is an augmented dataset with latitude and longitude added for each site.
Now, you can take a look at what are the coordinates for each site.
Above coordinates are just plain numbers that can not give you any intuitive insights about where are those launch sites. If you are very good at geography, you can interpret those numbers directly in your mind. If not, that's fine too. Let's visualize those locations by pinning them on a map.
We first need to create a folium Map
object, with an initial center location to be NASA Johnson Space Center at Houston, Texas.
We can show a blue circle and a popup label for the NASA Johnson Space Center.
TODO: Create and add folium.Circle
and folium.Marker
for each launch site on the site map
An example of folium.Circle:
folium.Circle(coordinate, radius=1000, color='#000000', fill=True).add_child(folium.Popup(...))
An example of folium.Marker:
folium.map.Marker(coordinate, icon=DivIcon(icon_size=(20,20),icon_anchor=(0,0), html='<div style="font-size: 12; color:#d35400;"><b>%s</b></div>' % 'label', ))
Now, you can explore the map by zoom-in/out the marked areas , and try to answer the following questions:
Are all launch sites in proximity to the Equator line?
Are all launch sites in very close proximity to the coast?
Also please try to explain your findings.
Task 2: Mark the success/failed launches for each site on the map
Next, let's try to enhance the map by adding the launch outcomes for each site, and see which sites have high success rates. Recall that data frame spacex_df has detailed launch records, and the class
column indicates if this launch was successful or not
Next, let's create markers for all launch records. If a launch was successful (class=1)
, then we use a green marker and if a launch was failed, we use a red marker (class=0)
Note that a launch only happens in one of the four launch sites, which means many launch records will have the exact same coordinate. Marker clusters can be a good way to simplify a map containing many markers having the same coordinate.
TODO: Create a new column in launch_sites
dataframe called marker_color
to store the marker colors based on the class
value
TODO: For each launch result in spacex_df
data frame, add a folium.Marker
to marker_cluster
From the color-labeled markers in marker clusters, you should be able to easily identify which launch sites have relatively high success rates.
TASK 3: Calculate the distances between a launch site to its proximities
Next, we need to explore and analyze the proximities of launch sites.
Let's first add a MousePosition
on the map to get coordinate for a mouse over a point on the map. As such, while you are exploring the map, you can easily find the coordinates of any points of interests (such as railway)
Now zoom in to a launch site and explore its proximity to see if you can easily find any railway, highway, coastline, etc. Move your mouse to these points and mark down their coordinates (shown on the top-right) in order to the distance to the launch site.
You can calculate the distance between two points on the map based on their Lat
and Long
values using the following method:
TODO: Mark down a point on the closest coastline using MousePosition and calculate the distance between the coastline point and the launch site.
TODO: After obtained its coordinate, create a folium.Marker
to show the distance
TODO: Draw a PolyLine
between a launch site to the selected coastline point
Your updated map with distance line should look like the following screenshot:
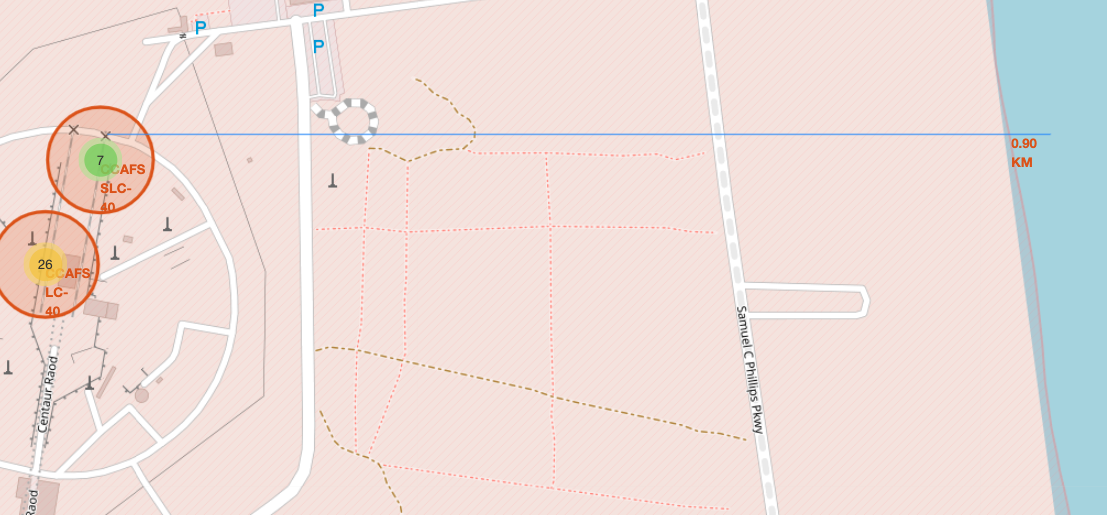
TODO: Similarly, you can draw a line betwee a launch site to its closest city, railway, highway, etc. You need to use MousePosition
to find the their coordinates on the map first
A railway map symbol may look like this:
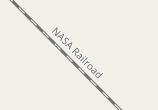
A highway map symbol may look like this:
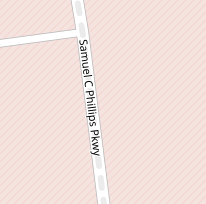
A city map symbol may look like this:
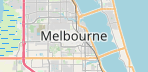
After you plot distance lines to the proximities, you can answer the following questions easily:
Are launch sites in close proximity to railways?
Are launch sites in close proximity to highways?
Are launch sites in close proximity to coastline?
Do launch sites keep certain distance away from cities?
Also please try to explain your findings.
Next Steps:
Now you have discovered many interesting insights related to the launch sites' location using folium, in a very interactive way. Next, you will need to build a dashboard using Ploty Dash on detailed launch records.
Authors
Other Contributors
Joseph Santarcangelo
Change Log
Date (YYYY-MM-DD) | Version | Changed By | Change Description |
---|---|---|---|
2021-05-26 | 1.0 | Yan | Created the initial version |
Copyright © 2021 IBM Corporation. All rights reserved.